WaveVR_CameraTexture¶
Contents |
Introduction¶
WaveVR_CameraTexture.cs is a C# wrapper to get access to the preview image from the Android camera or external camera (if one is available). You need to add permissions as shown below to access the Android camera in AndroidManifest.xml if you want to use the CameraTexture
<uses-permission android:name=”android.permission.CAMERA” />
WaveVR_CameraTexture provides several functions to get properties of native camera included
- isStarted
return true if native camera started.
- GetCameraImageType
return image type either WVR_CameraImageType_SingleEye or WVR_CameraImageType_DualEye. Suggest to check this before you decide how to display your image.
- GetCameraImageWidth
return width of camera image. 0 means camera is not started.
- GetCameraImageHeight
return height of camera image. 0 means camera is not started.
- getNativeTextureId
return native texture id of camera texture. 0 means camera is not started.
it also provides APIs to control native camera included
- startCamera
return true if camera is ready to start. The result of startCamera will update by StartCameraCompletedDelegate.
- stopCamera
call this API to stop native camera.
- updateTexture(uint textureId)
call this API with texture ID, WaveVR will update image content in which id you provided. After update texture is done by native, UpdateCameraCompletedDelegate will be called to notify.
Moreover, WaveVR_CameraTexture provides delegates to notify user if camera status updated. Developer is able to monitor delegates to proceed actions after camera status changes.
- StartCameraCompletedDelegate
StartCameraCompleted(bool result) is prototype and will be implemented by user who want to monitor camera start result and add it to StartCameraCompletedDelegate, WaveVR will notify user with result after camera start.
- UpdateCameraCompletedDelegate
UpdateCameraCompleted(uint nativeTextureId) is prototype and will be implemented by user who want to monitor camera update complete event. This function will be called if there is no newer data.
Resource¶
Script WaveVR_CameraTexture.cs is located under Assets/WaveVR/Scripts
Reference scene CameraTexture_Test is located under Assets/Samples/CameraTexture_Test/Scenes (in sample.package)
Sample script is located under Assets/Samples/CameraTexture_Test/Scripts (in sample.package)
How to Use¶
- In this sample, we use Android camera as a camera source. Add permissions to AndroidManifest.xml.

- Open the sample scene CameraTexture_Test.
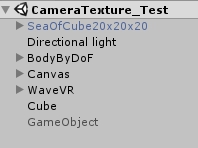
we add a cube in game hierarchy, and will update camera image on the cube.
- Look in Inspector of CameraTexture
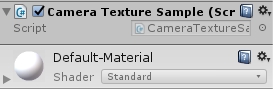
Source code¶
- start camera
WaveVR_CameraTexture.UpdateCameraCompletedDelegate += updateTextureCompleted;
WaveVR_CameraTexture.StartCameraCompletedDelegate += onStartCameraCompleted;
started = WaveVR_CameraTexture.instance.startCamera();
nativeTexture = new Texture2D(1280, 400);
textureid = nativeTexture.GetNativeTexturePtr();
meshrenderer = GetComponent<MeshRenderer>();
meshrenderer.material.mainTexture = nativeTexture;
- implement delegates to listen WaveVR_CameraTexture event.
void updateTextureCompleted(uint textureId)
{
Log.d(LOG_TAG, "updateTextureCompleted, textureid = " + textureId);
meshrenderer.material.mainTexture = nativeTexture;
updated = true;
}
void onStartCameraCompleted(bool result)
{
Log.d(LOG_TAG, "onStartCameraCompleted, result = " + result);
started = result;
}
- update camera texture
if (started && updated)
{
updated = false;
WaveVR_CameraTexture.instance.updateTexture((uint)textureid);
}