WaveVR_EventSystem¶
Contents |
Introduction¶
Unity provides Event System to receive input from standard I/O and communicate among different Game Objects.
In WaveVR, we provide several scripts and samples showing how to use Event System in a VR environment.
Documents¶
Developer can read the documents below to learn about the WaveVR Event System:
Gaze Input¶
If a VR environment does not have a controller, how does the user control items?
The answer is Gaze.
Gaze is sending events through the Event System to the center of the screen where a user is looking.
When Game Objects receive events, corresponding actions are taken.
Controller Input¶
In this document, WaveVR shows how to use the Event System on multiple controllers.
Advanced Usage¶
Developer might have questions after reading documents GazeInputModule and WaveVR Controller Input Module.
Eq.
- Is there an easier way to use EventSystem?
- Can I use the Gaze & Controller concurrently?
- Can I enable/disable Gaze or Controller Input Module in runtime?
- What does the scene MixedInputModule_Test in Assets/Samples/ControllerInputModule_Test demonstrate for?
All above questions have answers.
WaveVR provides a very easy way to use EventSystem for Gaze & Controller concurrently introduced below:
Sample¶
- Open the sample scene MixedInputModule_Test. It is a little bit different from ControllerInputModule_Test.
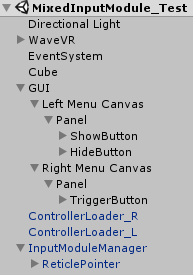
- Developer can see the InputModuleManager. It is a prefab from Assets/WaveVR/Prefabs.
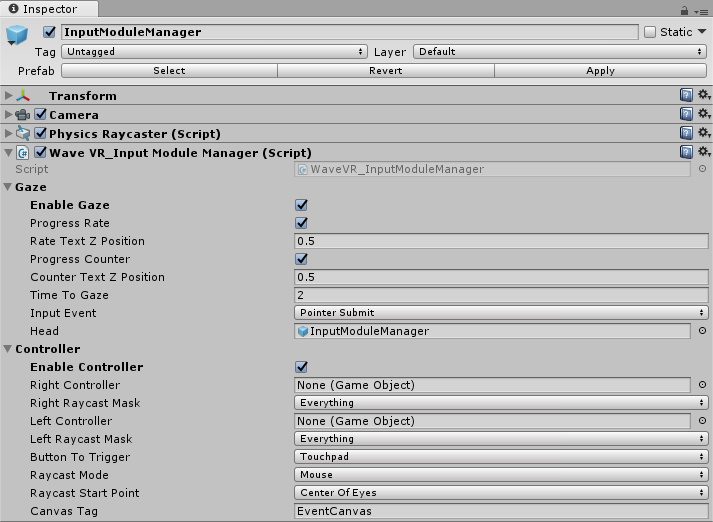
If developer already read the documents GazeInputModule and WaveVR Controller Input Module , parameters of WaveVR_InputModuleManager are the same.
If not, don’t worry, just keep the default settings and check the options you want: Enable Gaze or Enable Controller.
Developer can select one or both of them but only one input module can take effect.
If both of Enable Gaze and Enable Controller are selected, WaveVR_InputModuleManager will detect the controller connection, and then, enable controller input module if controller(s) is connected or enable gaze input module otherwise.
You can set up the Raycast Mode and the Raycast Start Point by the settings of InputModuleManager. Please refer to ControllerInputMode_Test for demonstration. Both settings could not work during runtime of preview, this setting can only take effect at beginning.
The raycast mode has three options, Mouse, Fixed and Beam.
Mouse mode: You can trigger object event through the pointer of controller.
Fixed mode: The beam length is fixed and you can trigger object event through the intersection of controller beam and object.
Beam mode: The location of controller pointer will move to the intersection of controller beam and object, you can trigger object event through the intersection of controller beam and object.
The raycast start point means the location of raycast event camera of mouse mode, there are three options (Center Of Eyes, Left Eye and Right Eye).
Also, this prefab plays the role as Head and PhysicRaycaster.
Note: Enable Gaze and Enable Controller value can be set in runtime.
The ControllerLoader_R and ControllerLoader_R are already introduced in WaveVR Controller Input Module .
They are prefab from Assets/WaveVR/Prefabs used to load controller. Developer needs to select Which Hand and Tracking Method.
3DOF means tracking rotation only. 6DOF means tracking rotation and position.
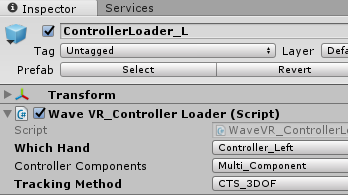
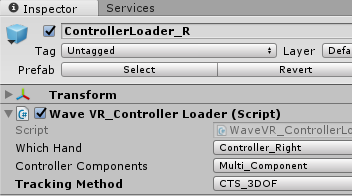
In WaveVR Controller Input Module , WaveVR_AddEventSystemGUI.cs is introduced.
It is used to mark the canvas which can receive event from EventSystem.
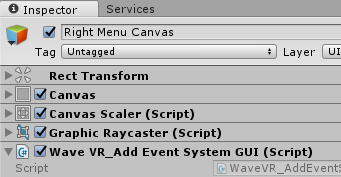
Last, set your own Event Handler to handle events .
WaveVR provides a sample script WaveVR_EventHandler in Assets/WaveVR/Extra/EventSystem.
Developer can simply copy the script and overwrite APIs in region #region override event handling function.
#region override event handling function
public void OnPointerEnter (PointerEventData eventData)
public void OnPointerExit (PointerEventData eventData)
public void OnPointerDown (PointerEventData eventData)
public void OnBeginDrag(PointerEventData eventData)
public void OnDrag(PointerEventData eventData)
public void OnEndDrag(PointerEventData eventData)
public void OnDrop(PointerEventData eventData)
public void OnPointerHover (PointerEventData eventData)
In this sample, we use it in Cube.
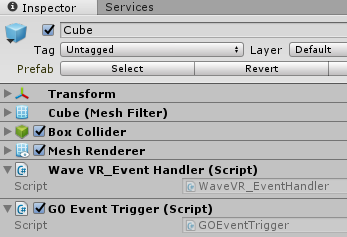
Now, all components needed in EventSystem are ready:
- InputModule (gaze & controller selected from WaveVR_InputModuleManager).
- Head component (prefab InputModuleManager).
- Controller component (loaded by ControllerLoader).
- Physic raycaster (contained in head and controller component).
- Graphyc raycaster (specified by WaveVR_AddEventSystemGUI)
- Event handler (override WaveVR_EventHandler)
Summary¶
So, in a scene without EventSystem, now developer knows how to enable EventSystem. Let’s have a practice from beginning.
Before start up, we need to prepare a scene, so creating a new scene as below:
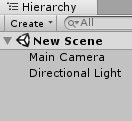
Consider a scene always has 3D physical object(s), add a simple cube:
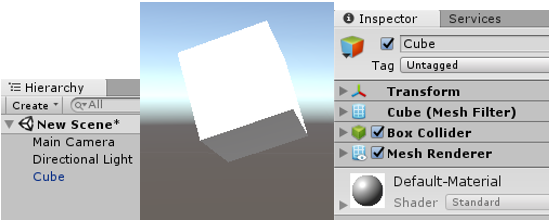
Consider a scene also has GUI(s), add a simple button:
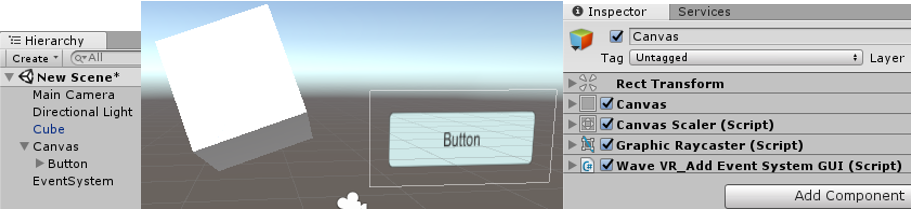
When adding a Button, Canvas and EventSystem will also be added automatically.
Note: must change the Render Mode of Canvas to World Space:
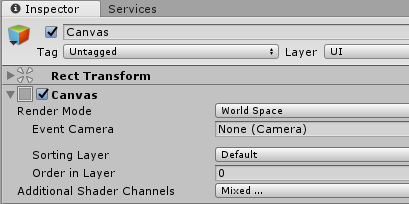
Initial scene is ready now, let’s use EventSystem step-by-step:
- Remove Main Camera, drag WaveVR from Assets/WaveVR/Prefab - for enabling WaveVR rendering.
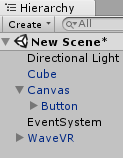
- Drag ControllerLoader prefab from Assets/WaveVR/Prefabs and choose options - for using a controller.
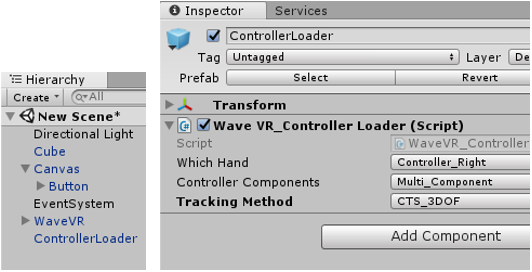
- Drag InputModuleManager prefab from Assets/WaveVR/Prefabs and select gaze or/and controller.
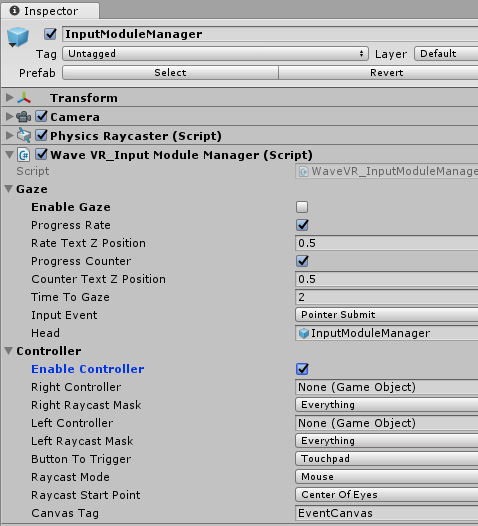
- Add component WaveVR_AddEventSystemGUI to GUI (Canvas of Button).
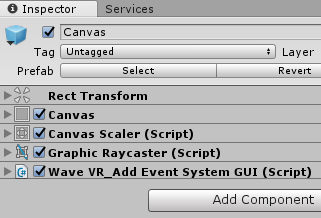
- Add component WaveVR_EventHandler to Cube
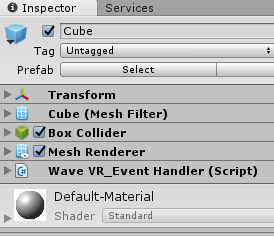
Done. Now developer can play scene in editor mode to see the effect.
Hint: use Right-Alt + mouse to controll the controller in scene.
Knack¶
How to set Enable Gaze or Enable Controller value in runtime?
Add code to your script:
WaveVR_InputModuleManager.Instance.Gaze.EnableGaze = true; // or false
WaveVR_InputModuleManager.Instance.Controller.EnableController = true; // or false