WaveVR_IME¶
Contents |
Introduction¶
We provide an IME sample in Assets/Misc/IME.
Open the sample scene IME_Test in Scenes.
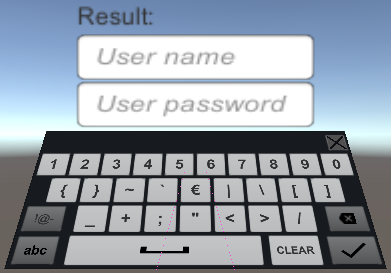
This sample contains:
- 1 Canvas with 1 Text and 2 InputField
- 4 Keyboard Canvas
Canvas: Includes 1 Text and 2 InputField. In InputField, we put two scripts: MyInputField and InputFieldManager.
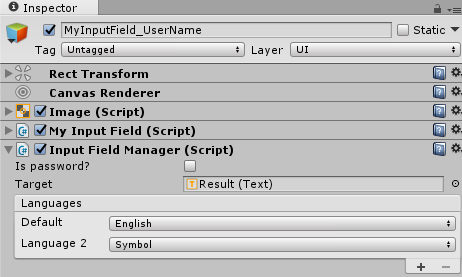
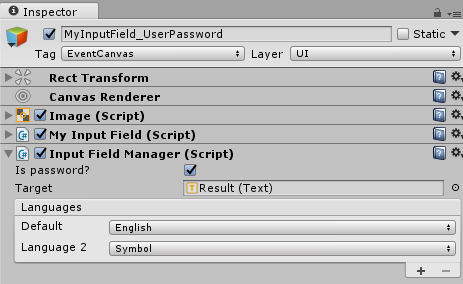
4 keyboard canvases: Alphabet, CapitalAlphabet, PunctualtionNumber and ShftPunctualtionNumber. Each keyboard has the KeyboardScript and specifies its own Type.
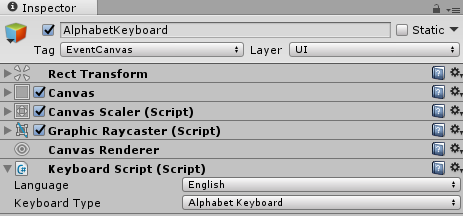
Inside the keyboard canvas, there are many Button GameObjects and each of them has the EventButton component.
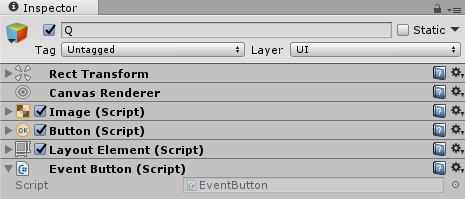
There are five canvases (Canvas and 4 keyboards) and all have the EventCanvas Tag.

When clicking on different buttons on the keyboard, different actions can be done:
- text buttons: show the related text on InputField.
- Clear or Backspace button: text on InputField is erased.
- Submit button: text on InputField is passed to Text in Canvas.
- Change / Shift button: change keyboard change.
- Close button: close keyboard.
You can run this sample in Editor mode and on an Android device.
Before building the APK, you must confirm the Android plugins in Assets/Plugins/Android.
If a developer wants to use the default Unity Android settings, it is recommended to remove the Assets/Plugins/Android folder.
To use IME in VR, we provided scripts and prefabs in Assets/Samples/IME_Test to integrate with WaveVR.
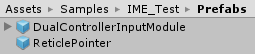

How to Use¶
- Drag the prefab DualControllerInputModule into IME_Test and disable the original Camera in the scene:
(DualControllerInputModule: this is a prefab from the another sample (refer to ControllerInputModule_Test) used to get input from the controllers or Gaze.)
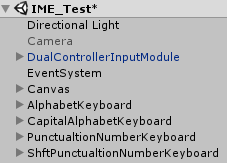
- Add the module WaveVR_CombinedInputModule into EventSystem and set up all columns:
(Script WaveVR_CombinedInputModule is introduced in Script section)
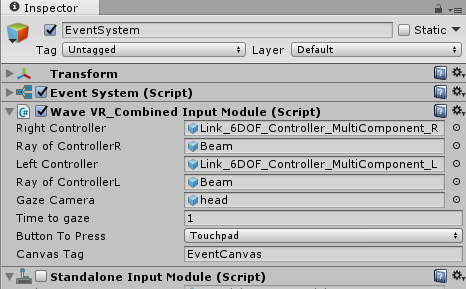
Note: the value Canvas Tag column must be set to EventCanvas equivalent to

- Make sure that Assets/Plugins/Android is already imported from wavevr.unitypackage
Script¶
Script hierarchy:
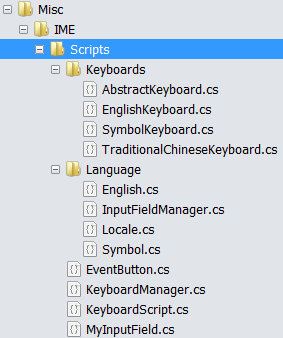
Keyboards: manage the instances of the keyboard of different languages.
Language: manage the function of the keyboard of different languages.
In previous sections, developer can see 5 scripts: WaveVR_CombinedInputModule, InputFieldManager, MyInputField, KeyboardScript and EventButton.
Also, there is an another script KeyboardManager used to manage the KeyboardScript.
WaveVR_CombinedInputModule: this script combines the gaze and controller input feature.
It is the mix of WaveVR_ControllerInputModule and GazeBasicInputModule. It has 8 input parameters:
- Right Controller
- Ray of ControllerR: ray of right controller used to point at object.
- Left Controller
- Ray of ControllerL: ray of left controller used to point at object.
- Gaze Camera: camera used for gaze
- Time to gaze: time in seconds to trigger gaze input. Default 1 second means when looking at the same object for 1 second, an event will be sent to object.
- Button To Press: specify the controller button used to trigger event. Default Touchpad means when pressing touchpad, an event will be sent.
- Canvas Tag: the Tag used to recognize Canvas which WaveVR_CombinedInputModule can take effect.
InputFieldManager: this script contains the actions of InputField and register onClick listener of keyboard button.
When receiving Submit or PointerClick event,
- The keyboards used for InputField will be initialized.
- Keyboards of each locale will be set up.
- First specified language will be enabled.
public void OnSubmit (BaseEventData eventData)
{
if (!initialized)
{
InitializeKeyboards ();
if (htLocaleArray.Count > 0)
{
foreach (KeyboardLanguage _kl in htLocaleArray.Keys)
{
curLocale = _kl;
break;
}
}
}
SetupKeyboards ();
KMInstance.DeactivateAllKeyboards ();
EnableLocale (curLocale);
}
Since different keyboard buttons have different actions, different function should be registered as onClick, different from Button.
E.g. Alphabet Keyboard of the English language
private void SetAlphabetKeyboard(GameObject _keyboard)
{
Button[] btns = _keyboard.GetComponentsInChildren<Button> ();
if (btns != null)
{
for (int i = 0; i < btns.Length; i++)
{
btns [i].onClick.RemoveAllListeners ();
switch (btns [i].name)
{
case "Clear":
btns [i].onClick.AddListener (OnClearText);
break;
case "Submit":
btns [i].onClick.AddListener (OnSubmitText);
break;
case "Change":
btns [i].onClick.AddListener (OnAlterKeyboard);
break;
case "Shift":
btns [i].onClick.AddListener (OnShiftKeyboard);
break;
case "Backspace":
btns [i].onClick.AddListener (OnBackspaceText);
break;
case "Space":
btns [i].onClick.AddListener (() => OnInputText (" "));
break;
case "Close":
btns [i].onClick.AddListener (OnCloseKeyboard);
break;
default:
string s = btns [i].name;
btns [i].onClick.AddListener (() => OnInputText (s));
break;
}
}
}
}
MyInputField: this script overwrites the default Unity InputField.
KeyboardScript: register the kayboard canvas with specified type to KeyboardManager as a singleton to be used across multiple InputField.
KeyboardManager: manages all registered keyboards.
Because there are multiple InputField that use the Alphabet Keyboard, we do not need to create Alphabet Keyboard instance for each InputField
We can easily get the instance of Alphabet Keyboard:
KeyboardManager.Instance.GetKeyboard(KeyboardManager.KeyboardType.AlphabetKeyboard);
EventButton: besides onClick, we need more features for Button, e.g. hover effect. This script handles events from EventSystem.