WaveVR_Controller¶
Contents |
Introduction¶
WaveVR_Controller.cs provides the developer an interface to access the controller.
By using WaveVR_Controller, the developer can easily get the button state & axis, pose of the controller.
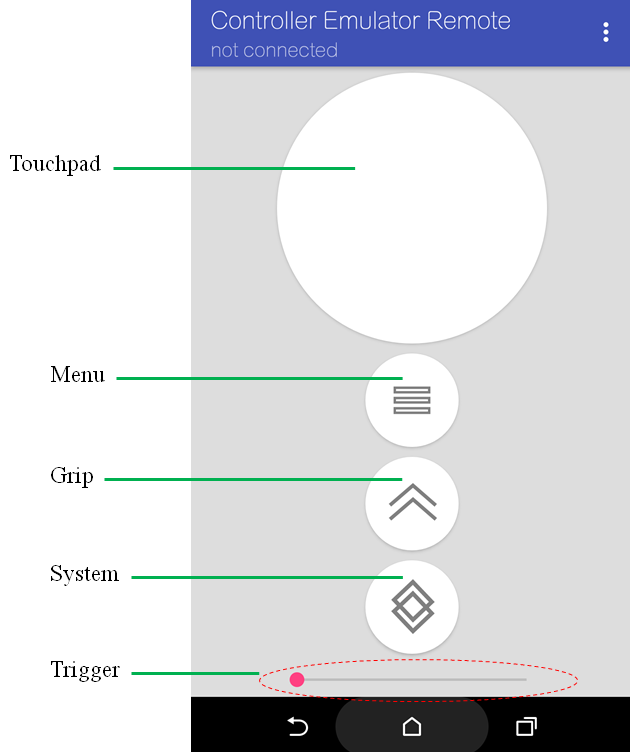
Resources¶
Sample scene Controller_Test is located in Assets/Samples/Controller_Test/Scenes
Sample script ControllerTest.cs is located in Assets/Samples/Controller_Test/Scripts
How to Use¶
- Open sample scene Controller_Test.
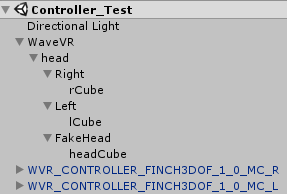
- Look at the Inspector of Right, Left and FakeHead, the sample script ControllerTest.cs is used. We put rCube, lCube or headCube to the Controlled Object that is controlled by Device.
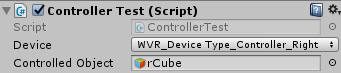
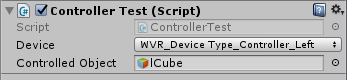
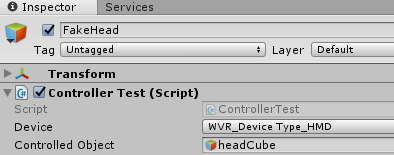
Sample Code¶
WaveVR_Controller is an API interface from which the events of the controller are received by specifying the Device.
By using WaveVR_Controller.Input(device), the developer can easily get device status:
if (WaveVR_Controller.Input(device).GetPressDown(buttonId))
{
// if button with buttonId is pressed down.
}
The developer can listen to a specified button by changing the buttonId:
WVR_InputId[] buttonIds = new WVR_InputId[] {
WVR_InputId.WVR_InputId_Alias1_Menu,
WVR_InputId.WVR_InputId_Alias1_Grip,
WVR_InputId.WVR_InputId_Alias1_DPad_Left,
WVR_InputId.WVR_InputId_Alias1_DPad_Up,
WVR_InputId.WVR_InputId_Alias1_DPad_Right,
WVR_InputId.WVR_InputId_Alias1_DPad_Down,
WVR_InputId.WVR_InputId_Alias1_Volume_Up,
WVR_InputId.WVR_InputId_Alias1_Volume_Down,
WVR_InputId.WVR_InputId_Alias1_Touchpad,
WVR_InputId.WVR_InputId_Alias1_Trigger,
WVR_InputId.WVR_InputId_Alias1_Bumper,
WVR_InputId.WVR_InputId_Alias1_System
};
In this sample, we put a cube that will appear / disappear when the device button is pressed / unpressed.
Get the pose of device:
WaveVR_Controller.Input (device).transform
Get the device connection status:
WaveVR_Controller.Input (device).connected