WaveVR_ControllerLoader¶
Old version (2.0.25 and before): here
Contents |
Introduction¶
WaveVR_ControllerLoader is a generic controller loader which will dynamically load the controller model and its behavior through settings and runtime environment variables. The developer doesn’t need to redesign the controller model to support another device. The developer only needs to import the newer controller model and WaveVR will initialize the proper model when a different device is used. Precondition is following rules by WaveVR, please refer to How to make a controller model
Controller loader provides many settings for developer to select. The settings include:
- Which Hand
Hand assignment, it is either right-hand or left-hand.
- Controller Components
This means how many components are available in the controller model. It is either one or multiple (default) components. If you need to have a response for each button, please use multiple components.
- Tracking Position and Rotation
Developer can select options.
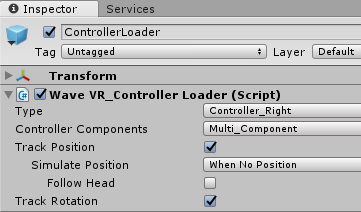
If Track Position is checked, Simulate Position checkbox will show.
If Simulate Position is selected as When No Position or Force Simulation, Follow Head checkbox will show.
Follow Head means that when controller is put (on table or whatever), controller should follow head movement or not in scene.
Note: the head position which controller follows is localPosition, not world position. Refer to Pose Tracker
- Indicator
Theremore, WaveVR_ControllerLoader will show indicator of controller model if the model supports, and provides following parameters to let developer customize your model:
Basic setting:
- Overwrite Indicator Settings
Overwrite setting of controller model or not, default value is off. This value set to true to activate following settings.
- Show Indicator
Enable indicator feature, default value is enable but requirement is controller model support.
- Hide Indicator when roll angle > 90
Hide indicator if controller roll angle is more than 90. default is true.
- Show Indicator Angle
Show indicator when controller pitch angle, default value is 30.
Line customization:
- Line Length
Line length between button and indicator, default value is 0.03f and range is 0.01f - 0.1f.
- Line Start Width
Starting width of line, default value is 0.0004f and range is 0.0001f - 0.1f.
- Line End Width
Ending width of line, default value is 0.0004f and range is 0.0001f - 0.1f.
- Line Color
Line color, default value is white.
Text customization (This used textMesh of Unity):
- Text Character Size
Non-chinese character size of text, default value is 0.08f,range is 0.1f - 0.2f.
- Chinese Character Size
Chinese character size of text, default value is 0.07f,range is 0.1f - 0.2f.
- Text Font Size
Font size of text, default value is 100f,range is 50 - 200f.
- Text Color
Text color, default value is white.
Button indication:
Developer uses a list to show button indication, each item includes
- Key Type
WaveVR_ControllerLoader supports button include
- Trigger
- Touchpad
- Bumper
- App
- Home
- Volume
- Alignment
Indication should present on which side, default value is right.
- Indication offset
Indication default computed by origin of game object, developer might use this offset value to adjust position.
- Indication text
The content used to present, developer might use keyword “system” to show content as multi-language. How to use multi-language, please refer to WaveVR_Resource
- Follow Button Rotation
The indication follows button’s rotation, default is false;
Finally, controllerLoader will broadcast CONTROLLER_MODEL_LOADED event with device type and controller instance to all when the controller model loads successfully. Please monitor this event if you need to get the controller instance to proceed or customize. Moreover, controllerLoader will broadcast CONTROLLER_MODEL_UNLOADED event when controller is disconnected.
Resource¶
Prefab ControllerLoader is located in Assets/WaveVR/Prefabs
Script WaveVR_ControllerLoader.cs is located in Assets/WaveVR/Scripts
Reference scene SeaOfCubeWithTwoHead is located in Assets/Samples/SeaOfCube/Scene (in sample.unitypackage)
Generic controller models are located in Assets/WaveVR/Resources/Controller (please refer to Generic Controller Model)
Link-like controller models are located in Assets/ControllerModel/Link (please refer to Link Controller Model)
Finch-like controller models are located in Assets/ControllerModel/Finch (please refer to Finch Controller Model)
How to Use¶
Prefab usage¶
Since there are so many options, we won’t demonstrate thoese photo by photo.
Usage is very simple:
- Drag the prefab ControllerLoader into your scene and rename to whatever you like.
- Select Which Hand.
- Keep Controller Components as Multi_Component.
- Select position and/or rotation tracking options. Already described above in Introduction part.
How to monitor CONTROLLER_MODEL_LOADED/UNLOADED event¶
Broadcast event is activated after WaveVR is initialized and only works on the device.
void OnEnable()
{
WaveVR_Utils.Event.Listen(WaveVR_Utils.Event.CONTROLLER_MODEL_LOADED, fun1);
WaveVR_Utils.Event.Listen(WaveVR_Utils.Event.CONTROLLER_MODEL_UNLOADED, fun2);
}
private void fun1(params object[] args)
{
var _dt = (WVR_DeviceType)args[0];
var go = (GameObject)args[1];
}
private void fun2(params object[] args)
{
var _dt = (WVR_DeviceType)args[0];
}
If you want to know when a controller is loaded in play mode, implement WaveVR_ControllerLoader.onControllerModelLoaded delegate. See the example below. The go parameter is a controller instance generated by the controller loader.
public delegate void ControllerModelLoaded(GameObject go);
void OnEnable()
{
#if UNITY_EDITOR
WaveVR_ControllerLoader.onControllerModelLoaded += controllerLoadedHandler;
#endif
}
void OnDisable()
{
#if UNITY_EDITOR
WaveVR_ControllerLoader.onControllerModelLoaded -= controllerLoadedHandler;
#endif
}
void controllerLoadedHandler(GameObject go)
{
Debug.Log("controllerLoadedHandler : " + go.name);
}