Tracked Device¶
Tracked Device provides the interface for the HMD and controller devices. All functions for tracking is defined in this class.
List |
Pose¶
There are rotation and translation data types that need to be defined on Pose struct.
Rotation¶
For rotation tracker data, define the orientation of the tracker and then represent it as a quaternion.
Pose pose = new Pose();
pose.rotation = new Quaternion();
//Design your tracker rotation. For each 10 degree angle
//rotation of your device tracker, it will be like:
w = w + Math.PI/18;
x = x + Math.PI/18;
y = y + Math.PI/18;
z = z + Math.PI/18;
pose.rotation.w = w;
pose.rotation.x = x;
pose.rotation.y = y;
pose.rotation.z = z;
Translation¶
For positional tracker data, define the vector of the tracker and then represent it as a translation.
Pose pose = new Pose();
pose.translation = new Vector3();
//Design your tracker translation. If your device tracker
//translation is linear, it will be like:
x = x + 0.001;
y = y + 0.001;
z = z + 0.001;
pose.translation.x = x;
pose.translation.y = y;
pose.translation.z = z;
AngularVelocity¶
For angular velocity in radians/s, define the vector of the tracker and then represent it as an AngularVelocity.
The angular velocity of the pose is in the axis-angle representation. The direction is the angle of rotation and the magnitude is the angle around that axis in radians/second.
Pose Pose= new Pose();
Pose.angularVelocity= new Vector3();
//Design your tracker AngularVelocity. If your device tracker
//AngularVelocity is linear, it will be like:
x = x + 0.001;
y = y + 0.001;
z = z + 0.001;
pose.angularVelocity.x = x;
pose.angularVelocity.y = y;
pose.angularVelocity.z = z;
updatePose() notifies the server that a tracked device’s pose has been updated.
updatePose(pose, System.nanoTime());
Button¶
The following four APIs should be done together and their order should be as follows:
- setButtonPress(true, ButtonId, System.nanoTime())
- setButtonTouch(true, ButtonId, System.nanoTime())
- setButtonPress(false, ButtonId, System.nanoTime())
- setButtonTouch(false, ButtonId, System.nanoTime())
The parameter of buttonId is WVR_InputId.
Note
Whether the server is connected to the device service or not, the button data should always be sent to the server.
Recenter
onRecenter() function will be called when recenter is active.
- If device of VR kits supports HW-recenter, the developer should set the Attribute for the Recenter returned value.
- Attr_IsHWRecenter_Bool = 10110 , ask whether support HW recenter.
- For software (SW) recenter case, the developer doesn’t need to do any operation for SW recenter in device service/driver. Runtime will handle controller “zero” orientation for it.
- For below cases, please consider to use software (SW) recenter for your controller.
- VR kits supports recenetr controller only case. This case needs runtime to make yaw angle of controller align yaw angle of HMD.
- HMD and controller are not using the same (manufacturer’s) tracking reference solution.
- For below cases, please consider to use software (SW) recenter for your controller.
Recenter button could send event to the device service through the server when button is pressed.
@Override
public void onRecenter() {
Debug.Log("get Recenter Notification");
}
set 3DoF/6DoF scene
on6DofScene(boolean is6DoF) function will be called when the scene is switched to 3DoF or 6DoF.
- Parameter:
- is6DoF: it is a boolean parameter which specifies the scene is 3DoF or 6DoF now. When the scene is changing, on6DofScene() will be used and this parameter will be inputted.
- True: Now the scene is switched to 6DoF. False: Now the scene is switched to 3DoF.
@Override
public void on6DofScene(boolean is6DoF) {
if (is6DoF) {
Debug.Log("the scene is 6DoF now");
} else {
Debug.Log("the scene is 3DoF now");
}
}
notifies tracking mode changed to 3DoF or 6DoF
onTrackingModeChanged(int mode) function will be called when the setting AP switched device tracking mode to 3DoF or 6DoF.
- Parameter:
- mode: It is a int parameter which specifies the tracking mode is 3DoF or 6DoF now. Device should change its tracking mode if supported.
- WVR.TrackingMode.TrackingMode_3DoF: switched to 3DoF tracking mode. WVR.TrackingMode.TrackingMode_6DoF: switched to 6DoF tracking mode.
@Override
public void onTrackingModeChanged(int mode) {
switch (mode) {
case WVR.TrackingMode.TrackingMode_3DoF:
// switch device to 3DoF tracking mode
break;
case WVR.TrackingMode.TrackingMode_6DoF:
// switch device to 6DoF tracking mode
break;
}
}
Bumper Button
If there is a trigger on your controller but it doesn’t support analog function,
use Bumper Button to update the button state. Do not use the trigger.
Virtual D-Pad on Touchpad
The Virtual D-Pad button would be implemented on Touchpad by default to make up no physical D-Pad on controller. The setup of Virtual D-Pad is as follows:
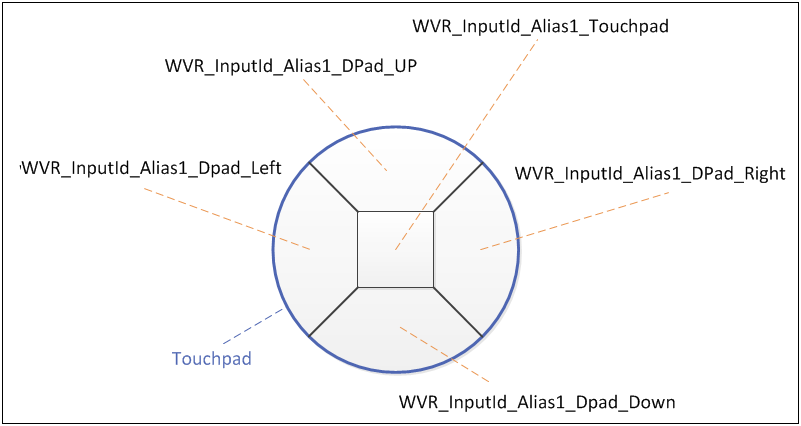
See also WVR_InputId enum type on Types in wvr.h page.
Analog¶
The analog is divided into two components: trigger and touchpad. updateAnalog() is used to transmit the trigger or touchpad data.
You determine the value for the Triggeraxis.
PointF Triggeraxis = new PointF();
When the button WVR.InputId_Alias1_Trigger is pressed, only the Triggerarix.x analog value will change. The Triggerarix.x value should be between 0~1.
updateAnalog(WVR.InputId_Alias1_Trigger, Triggeraxis, System.nanoTime());
When the button WVR.InputId_Alias1_Touchpad is touched, the Triggerarix.x and Triggerarix.y analog values will both change. The Triggerarix.x value when there are horizontal swipes should be between -1~1. The Triggerarix.y value when there vertical swipes should be between -1~1.
updateAnalog(WVR.InputId_Alias1_Touchpad,Triggerarix, System.nanoTime());
Swipe Motion on Touchpad
The holding conditions of the swipe motion (from start touched point to end touched point) are as follows:
- 1.Valid swipe distance:
- The valid swipe distance on the touchpad should be greater than 0.2. (based on the diameter of Touchpad is 2)
- 2.Valid swipe angle:
- Horizontal: 45 degree > angle > -45 degree
- Vertical: 135 degree >= angle >= 45 degree